main.js (3830B)
1 (function() { 2 const md = ` 3 # Hello, World. 4 ------ 5 First off *why* did I build this when their are so many other markdown editors out there?... 6 __Vim__ ftw that's why! And no other markdown editors out there have my must have vim keymapping \`imap kj <Esc>\` 7 now without further ado 8 Here is a list of the stuff you can do in markdown. 9 10 ## First the easy stuff 11 12 * Lists! 13 * in bullet 14 * form 15 16 ### Also... 17 18 1. numbered lists! 19 2. in numerical 20 3. form 21 22 #### And then there was this 23 24 1. Numerical bullets 25 * with sub bullets 26 27 ##### link all the things 28 29 [Links!](http://www.google.com) Links to all sorts of stuff 30 31 ###### And finally 32 33 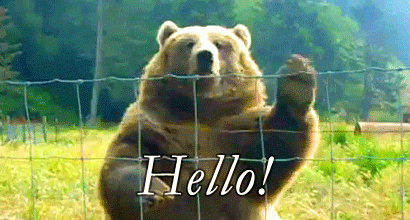 34 35 But also don't forget... 36 37 \`\`\`\javascript 38 (function() { 39 return Math.floor(Math.random() * 100); 40 })(); 41 \`\`\`\ 42 43 Also this 44 45 \`\`\`html 46 <!doctype html> 47 <html lang="en-us"> 48 <head> 49 <title>My Website</title> 50 </head> 51 <body></body> 52 </html> 53 \`\`\` 54 55 And one of my personal favorites __tables__ 56 57 Simple | Markdown | Table 58 --- | --- | --- 59 *looks* | \`pretty\` | **good** 60 1 | 2 | 3 61 62 And so on so forth. 63 64 Happy writing in **VIM** mwahahaha! 65 `; 66 67 if (!window.localStorage.getItem('markdown') || !window.localStorage.getItem('markdown').replace(/\s/g, '')) { 68 window.localStorage.setItem('markdown', md) 69 } 70 71 const editor = CodeMirror(document.querySelector('.js-input'), { 72 value: window.localStorage.getItem('markdown'), 73 mode: "javascript", 74 lineWrapping: true, 75 keyMap: 'vim', 76 theme: 'the-matrix' 77 }); 78 79 if (editor) { 80 CodeMirror.Vim.map('kj', '<Esc>', 'insert') // to use kj as <Esc> also 81 82 hljs.initHighlightingOnLoad(); 83 84 if (window.localStorage.getItem('markdown')) { 85 editor.value = window.localStorage.getItem('markdown'); 86 document.querySelector('.js-content').innerHTML = marked(window.localStorage.getItem('markdown')); 87 } 88 else { 89 document.querySelector('.js-content').innerHTML = editor.getValue(); 90 } 91 document.querySelector('.js-input').addEventListener('keyup', event => { 92 const transformed = marked(editor.getValue()); 93 document.querySelector('.js-content').innerHTML = transformed; 94 for (let i = 0; i < document.getElementsByTagName('code').length; i++) { 95 hljs.highlightBlock(document.getElementsByTagName('code')[i]); 96 } 97 window.localStorage.setItem('markdown', editor.getValue()); 98 }); 99 100 document.querySelector('.CodeMirror-vscrollbar').addEventListener('scroll', event => { 101 setTimeout(() => { 102 if ( document.querySelector('.js-content').parentElement.parentElement.scrollHeight - editor.getScrollInfo().top <= 1000 ) { 103 document.querySelector('.js-content').parentElement.parentElement.scrollTop = 1000000; 104 } 105 else { 106 document.querySelector('.js-content').parentElement.parentElement.scrollTop = editor.getScrollInfo().top; 107 } 108 }, 250) 109 }); 110 } 111 112 document.querySelector('.js-copy').addEventListener('click', event => { 113 const textarea = document.createElement('textarea'); 114 textarea.innerHTML = window.localStorage.getItem('markdown'); 115 const textToCopy = document.querySelector('textarea'); 116 document.querySelector('.js-input').appendChild(textarea); 117 textarea.focus(); 118 textarea.select(); 119 120 try { 121 document.execCommand('copy'); 122 } 123 catch (err) { 124 alert('Copy not supported'); 125 } 126 finally { 127 document.querySelector('.js-input').removeChild(textarea); 128 } 129 }); 130 131 })();